 |
 |
View previous topic :: View next topic |
Author |
Message |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Mon Mar 11, 2024 8:25 am |
|
|
Can you give an example of what you say in code? |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19238
|
|
Posted: Mon Mar 11, 2024 8:46 am |
|
|
You really do need to program for yourself. However using a totally different
approach:
Code: |
rom unsigned int8 wi[] = {
0x00,0x08,0x00,0x00,0x00,0x00,0x14,0x00,0x00,0x00,0x00,0x12,0x00,0x00,0x00,0x00,
0x21,0x00,0x00,0x00,0x00,0x21,0xe0,0x00,0x00,0x01,0x21,0xa0,0x00,0x00,0x01,0x21,
0xa0,0x00,0x00,0x01,0x21,0x90,0x00,0x00,0x81,0x20,0x90,0x00,0x00,0x81,0x10,0x90,
0x00,0x00,0x81,0x08,0x90,0x00,0x00,0x82,0x04,0x90,0x00,0x00,0x82,0x02,0x50,0x00,
0x00,0x02,0x01,0x60,0x00,0x00,0xc4,0x01,0xe0,0x00,0x00,0x38,0x02,0x10,0x01,0x00,
0x00,0x04,0x08,0x01,0x00,0x00,0x08,0x04,0x06,0x18,0x00,0x08,0x02,0x18,0x06,0x00,
0x30,0x01,0xe0,0x01,0x00,0xc0,0x00,0x00,0x00
}; //Must be rom not const
/* Function to accept x,y start coordinate, width and height of a pixel
based image stored in ROM (pointer passed), and draw this to the OLED*/
void SSD1306_ROMBMP(unsigned int16 x, unsigned int16 y, unsigned int16 width, unsigned int16 height, rom unsigned int8 *bitmap)
{
unsigned int16 row, column;
unsigned int16 row_length; //ROM row length rounded up to 8bits
unsigned int8 the_byte; //byte from the array
unsigned int8 bit_mask; //bit mask to reduce need for bit accesses
unsigned int16 location;
//Now row_length needs to be the nearest multiple of 8 above the
//number of columns (width) to give where stuff is in the array.
row_length=((width/8)+1)*8;
//Loop through the image rows, pulling data from the rom array
for (row=y;row<height+y;row++)
{
//Now loop through the columns
column = x;
do
{
//Now calculate byte number needed in the array
location = ((row-y)*row_length)+(column-x);
//now to reduce the number of array accesses store the byte
the_byte=bitmap[location/8]; //Fetch once for every eighth bit.
bit_mask=1;
//handle all the bits in this byte
do
{
if (the_byte & bit_mask)
oled_Pixel(column, row,1);
else
oled_Pixel(column, row,0);
bit_mask*=2; //compiler will code this as a rotation
//However will wrap to be 0 when 128 is multiplied.
//makes the looping easy.
column++;
} while (column<width+x && bit_mask!=0) ; //exits every 8bits
} while (column<width+x); //does the whole line
}
}
//
void oled_test_(void)
{ //oled test
i2c_init(true);
delay_ms(500);
//
Oled_Init();
oled_clearScreen();
oled_command(NORMALDISPLAY);
//
SSD1306_ROMBMP(20, 20, 37, 21, wi);
delay_ms(1000); //allow to be seen before returning
}
|
No guarantees on this, just typed from what I think you are trying to do. |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Mon Mar 11, 2024 9:20 am |
|
|
Thank you very much, I will test this code as soon as possible. |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Mon Mar 11, 2024 7:32 pm |
|
|
I thank the friends who helped me.
@Ttelmah the code my friend wrote worked, I made some changes to it. I am sending 3 different ROMs to the screen.
Code: | const unsigned int8 rom1[] = {
0x80,0x0f,0x00,0xe0,0x3f,0x00,0x78,0xf0,0x00,0x9c,0xcf,0x01,0xee,0xbf,0x03,0xf7,
0x78,0x07,0x3a,0xe7,0x02,0xdc,0xdf,0x01,0xe8,0xb8,0x00,0x70,0x77,0x00,0xa0,0x2f,
0x00,0xc0,0x1d,0x00,0x80,0x0a,0x00,0x00,0x07,0x00,0x00,0x02,0x00,0x00,0x00,0x00
};
//
const unsigned int8 rom2[] = {
0x00,0x00,0x00,0xf0,0xff,0x7f,0x08,0x00,0x80,0x08,0xdb,0xb6,0x0e,0xdb,0xb6,0x01,
0xdb,0xb6,0x01,0xdb,0xb6,0x01,0xdb,0xb6,0x01,0xdb,0xb6,0x01,0xdb,0xb6,0x0e,0xdb,
0xb6,0x08,0xdb,0xb6,0x08,0x00,0x80,0xf0,0xff,0x7f,0x00,0x00,0x00,0x00,0x00,0x00
};
//
const unsigned int8 rom3[] = {
0x38,0x00,0x44,0x40,0xd4,0xa0,0x54,0x40,0xd4,0x1c,0x54,0x06,0xd4,0x02,0x54,0x02,
0x54,0x06,0x92,0x1c,0x39,0x01,0x75,0x01,0x7d,0x01,0x39,0x01,0x82,0x00,0x7c,0x00
}; |
Code: | int8 rom_start = 0;
int8 row_select = 0;
//
void ROMBMP(unsigned int8 x, unsigned int8 y, unsigned int8 width, unsigned int8 height, rom unsigned int8 *bitmap)
{
unsigned int16 row, column;
unsigned int16 row_length;
unsigned int8 the_byte;
unsigned int8 bit_mask;
unsigned int16 location;
//
if(row_select == 0){
row_length=((width/8)+1)*8;
}
if(row_select == 1){
row_length=((width/8)+0)*8;
}
for (row=y;row<height+y;row++)
{
column = x;
do
{
location = ((row-y)*row_length)+(column-x);
if(rom_start == 1){
the_byte= rom1 [location / 8];}
//
if(rom_start == 2){
the_byte= rom2 [location / 8];}
//
if(rom_start == 3){
the_byte= rom3 [location / 8];}
//
bit_mask = 1;
do
{
if (the_byte & bit_mask)
oled_Pixel(column, row,1);
else
oled_Pixel(column, row,0);
bit_mask*=2;
column++;
} while (column<width+x && bit_mask!=0) ;
} while (column<width+x);
}
} |
Code: | int8 rom_flag1 = 0,rom_flag2 = 0,rom_flag3 = 0;
//
void oled_test_(){ //oled test
i2c_init(true);
delay_ms(500);
//
Oled_Init();
oled_clearScreen();
oled_command(NORMALDISPLAY);
//
if(rom_flag1 == 0){
rom_start = 1; //rom1 start
row_select = 0; //ilk grafik basma algoritması
ROMBMP(50, 20, 19, 16, rom1);}
//
if(rom_flag2 == 0){
rom_start = 2; //rom2 start
row_select = 1; //geri kalan grafik basma algoritması
ROMBMP(103, 0, 24, 16, rom2);}
//
if(rom_flag3 == 0){
rom_start = 3; //rom3 start
row_select = 1; //geri kalan grafik basma algoritması
ROMBMP(0, 0, 16, 16, rom3);}
//
char txt[5]; //sıcaklık ekrana basılıyor
sprintf(txt,"%02u",voltaj_ntc);
oled_text57(19, 0, txt, 2, 1); |
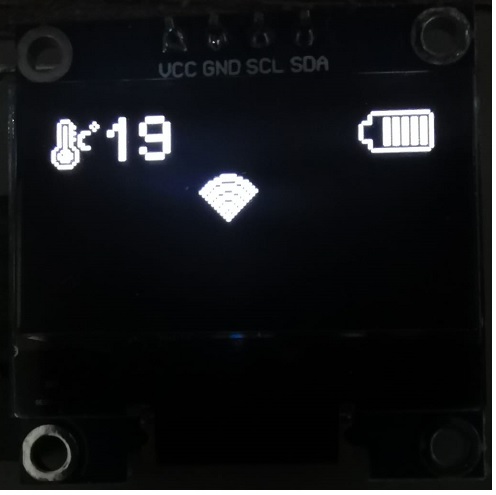
Last edited by bulut_01 on Tue Mar 12, 2024 7:41 am; edited 1 time in total |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9120 Location: Greensville,Ontario
|
|
Posted: Tue Mar 12, 2024 5:14 am |
|
|
Looks like Mr. T has done it again !!! Hurrah !!
curious like my cats ?
Why are row,column and location 16 bits when the GLCD is 128 x 64 bits ?
Jay |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Tue Mar 12, 2024 5:24 am |
|
|
temtronic wrote: |
Why are row,column and location 16 bits when the GLCD is 128 x 64 bits ?
Jay |
Best regards, I fixed it in 8 bit |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19238
|
|
Posted: Tue Mar 12, 2024 8:22 am |
|
|
I left them as 16bit, since the later version of the controller (11xx), supports
screens over 128 pixels wide. I suspect he drivers he has copied stuff
from, are written as 'universal' ones, supporting all versions of the chip.
On the PIC, it would result in faster maths, to use 8bit, if the screen is
never going to be any larger. |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Tue Mar 12, 2024 9:43 am |
|
|
8 bit fix after 16 bit testing phase. Is it possible to make progress bar with ccs? I'm patient, there is no progress bar library in ccs. |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9120 Location: Greensville,Ontario
|
|
Posted: Sun Mar 17, 2024 8:53 am |
|
|
'progress bar' is simple
1st decide on how big it will be ( say 80 bars long)
2nd decide on the each segment 'width',(say 10 bars wide)
3rd create 8 versions from 1 segment on to 8 on )
4th create a table (off,1,2,3,4,5,6,7,full)
5th cut code (a function) ,that reads the ADC and returns a number 0 to 7.use that number in a 'case'.
YOU will have to decide on the bars total length and resolution. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19238
|
|
Posted: Sun Mar 17, 2024 9:32 am |
|
|
My SSD1306 driver in the code library (designed for chips with small numbers
of pins and ROM), has a 'textbar' function, that generates bar graph.
This is done by programming text shapes and then just drawing the right
mix of these to give the graph. The code for this should be adaptable
to the later CCS driver. |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Sun Mar 17, 2024 10:23 am |
|
|
Oled ssd1306 refresh rate is very slow and very noticeable. Is there a faster way to do this ? |
|
 |
temtronic
Joined: 01 Jul 2010 Posts: 9120 Location: Greensville,Ontario
|
|
Posted: Sun Mar 17, 2024 10:34 am |
|
|
According to Google, refresh rate is up to 60Hz,
so I suspect
1) your code either hasn't configured the GLCD module correctly
2) your access code has delays in transferring the data to it.
3) maybe the I2C config is set slower than it should be ?
4) code is polling and not using interrupts ? |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Sun Mar 17, 2024 2:56 pm |
|
|
i2c Its configuration is set to 1 MHz, and I refresh the screen when some global variables change. It seems to me that it is quite slow during the refresh. The code of the screen draw algorithm related to oled_pixel was written by the friend here.
It uses the above rombmp algorithm while drawing. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19238
|
|
Posted: Mon Mar 18, 2024 1:35 am |
|
|
The SSD1306, does not support 1MHz I2C. It's maximum clock rate is
400KHz. From the data sheet in the I2C section. "Clock Cycle time min
2.5uSec".
That it is actually even working, suggests your I2C may not be clocking
as you think. You may well be using software I2C, possibly well below even
400KHz.
Post your processor setup and I2C setup.
Now that having been said, the 'bmp' code will be fairly slow. You are
pulling data using a pointer (inherently slow), and putting out a lot of bytes.
By the time you have sent the command, coordinates and the data bytes
perhaps 130 bytes each time. Each bmp shape (depending on it's size),
will probably take perhaps 1/50th second at 400kHz. If you are doing many
of these, then it could explain your slow redraw (not refresh).
You can run much faster by using SPI instead of I2C. Needs a couple more
pins, but gives 2.5* the supported rate.
Also, understand that the SSD does not support setting a pixel directly.
The 'set pixel' function, has to calculate what byte contains the pixel, read
this, then change the pixel in this, and write the byte back. Result a single
'set pixel' command will involve perhaps a dozen transfers on the I2C
bus. This is why my driver does not do this, it instead performs the
operation on a RAM buffer, then sends this whole buffer to the screen.
Given the speed the I2C has to run, and the number of bytes involved
using the set_pixel command, the times I say above go up by a factor
of a dozen, so each character will take perhaps 1/4 second. I2C again
makes this worse, since to read a byte,you have to set the bus to write,
write the address, then restart, set the bus to read, then read the byte.
About 50% more time than using another interface, and at a slower clock. |
|
 |
bulut_01
Joined: 24 Feb 2024 Posts: 62
|
|
Posted: Mon Mar 18, 2024 5:38 am |
|
|
i2c hardware setting has been made. What you say may be true that fast = 1000000 may change the algorithm in the draw drawing. I am not much of an expert on this subject. the screen is just slow.
#use fast_io(A)
#use fast_io(B)
#use fast_io(C)
//
#pin_select SCL1in = PIN_C3
#pin_select SDA1in = PIN_C4
#use i2c(master,I2C1, Fast=1000000, sda = PIN_C4, scl = PIN_C3 , STREAM = STREAM_24XX1025) |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|